1D setups
Advection (1D)
The following image shows the results of the 1D advection test for different hydro algorithms:
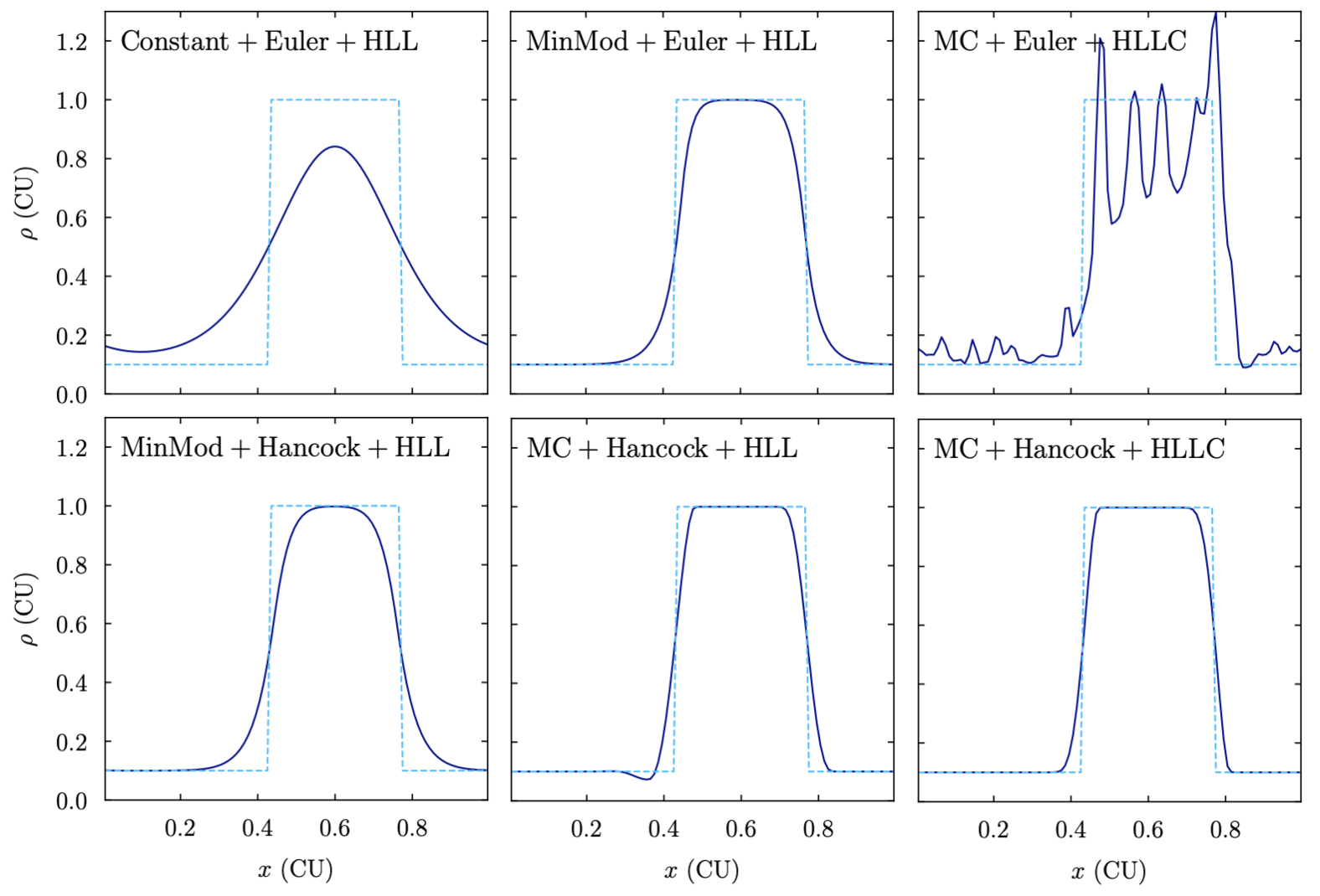
- class ulula.setups.advection_1d.SetupAdvection1D(shape='sine', unit_l=1.0, unit_t=1.0, unit_m=1.0)
1D advection test
This setup represents the perhaps simplest meaningful test of a hydro solver. An initial distribution in density is advected at a constant velocity across the domain. The shape should be preserved. This setup demonstrates
Stability and instability of schemes
Importance of choice of slope limiters and Riemann solvers.
- Parameters:
- shape: str
Initial shape of density that is being advected. Can be
sine
ortophat
.- unit_l: float
Code unit for length in units of centimeters.
- unit_t: float
Code unit for time in units of seconds.
- unit_m: float
Code unit for mass in units of gram.
- __init__(shape='sine', unit_l=1.0, unit_t=1.0, unit_m=1.0)
Soundwave
The image below shows the soundwave setup after a few wave periods:
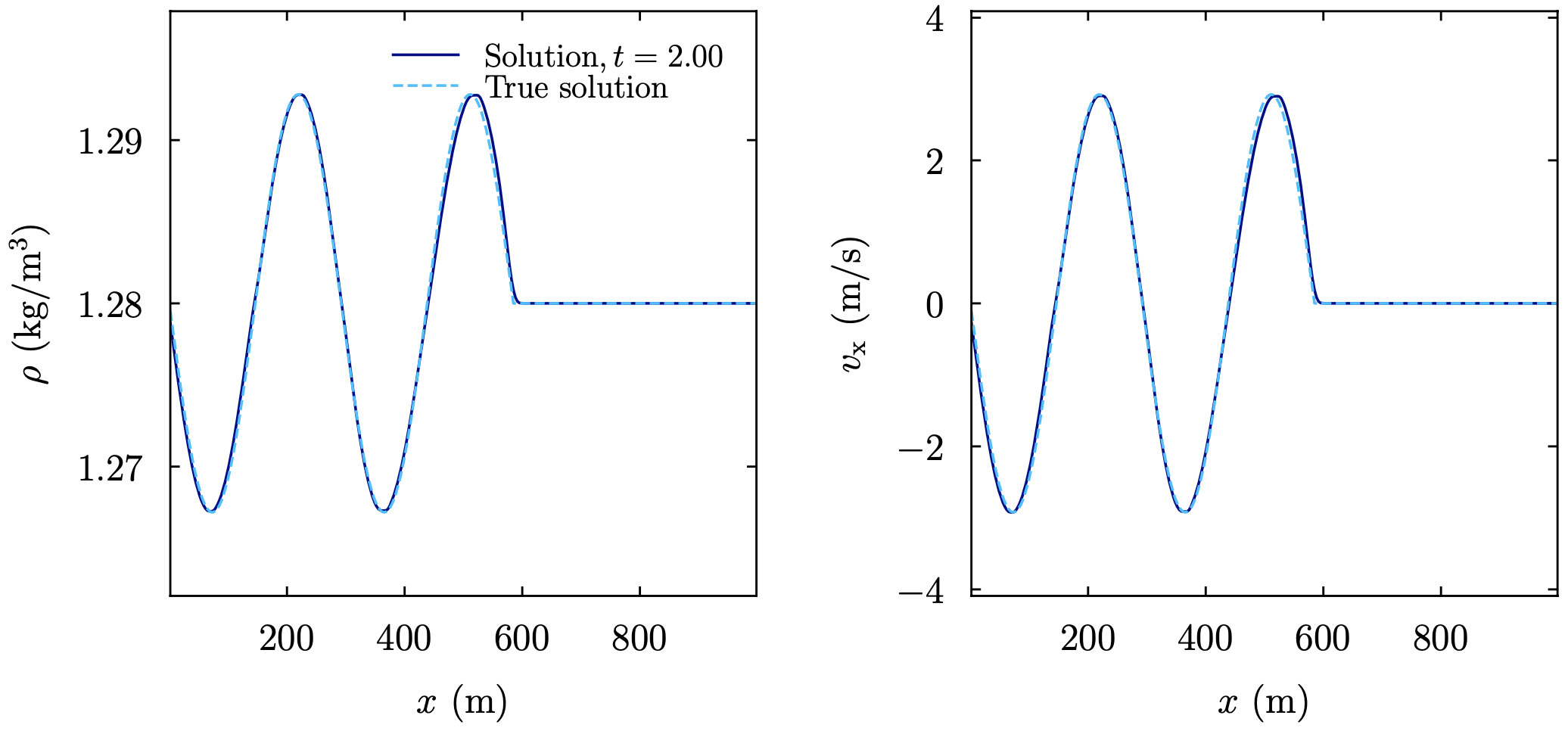
- class ulula.setups.soundwave.SetupSoundwave(L=100000.0, frequency=1.0, amplitude=0.01, eos_mode='ideal', eos_T_K=300.0, unit_l=100000.0, unit_t=1.0, unit_m=1000000000000.0)
Propagating sound wave
A sound wave is created at the left edge of the domain and travels to the right. We assume the density and adiabatic index of air, and either standard pressure or an isothermal EOS given by a particular temperature. This setup demonstrates
User-defined boundary conditions on the left
Outflow boundary conditions on the right
Difference between ideal and isothermal equations of state
Use of code units.
The true solution is based on linear theory, which only holds for small amplitudes. We observe wave steepening for large amplitudes.
- Parameters:
- L: float
The box size in cm.
- frequency: float
The frequency in Hertz with which a sound wave is created.
- amplitude: float
The relative amplitude by which the density is increased compared to the background.
- eos_mode: str
Can be
ideal
orisothermal
.- eos_T_K: float
Air temperature in Kelvin (if using isothermal EOS, ignored otherwise).
- unit_l: float
Code unit for length in units of centimeters.
- unit_t: float
Code unit for time in units of seconds.
- unit_m: float
Code unit for mass in units of gram.
- __init__(L=100000.0, frequency=1.0, amplitude=0.01, eos_mode='ideal', eos_T_K=300.0, unit_l=100000.0, unit_t=1.0, unit_m=1000000000000.0)
Shocktube
The image below shows the shocktube test for the default hydro solver:

- class ulula.setups.shocktube.SetupShocktube(gamma=1.4, x0=0.5, rhoL=1.0, rhoR=0.125, PL=1.0, PR=0.1, uL=0.0, uR=0.0, unit_l=1.0, unit_t=1.0, unit_m=1.0)
Shocktube problem
The shocktube setup represents a Riemann problem, i.e., a sharp discontinuity in a 1D domain. The default parameters represent a the Sod (1978) setup, which is a classic test for Riemann solvers. The sharp break in fluid properties causes a shock and a contact discontinuity traveling to the right and a rarefaction wave traveling to the left. This setup demonstrates:
Ability of hydro solver to handle strong shocks
Impact of slope limiting on the solution
Softening of sharp discontinuities at lower resolution.
The Sod problem can be solved analytically. The solution used here is based on lecture notes by Frank van den Bosch (pdf) and Susanne Hoefner (pdf). However, this solution may not work for all sets of input parameters.
- Parameters:
- gamma: float
The adiabatic index of the ideal gas.
- x0: float
The position of the discontinuity.
- rhoL: float
The density of the left state.
- rhoR: float
The density of the right state.
- PL: float
The pressure of the left state.
- PR: float
The pressure of the right state.
- uL: float
The velocity of the left state.
- uR: float
The velocity of the right state.
- unit_l: float
Code unit for length in units of centimeters.
- unit_t: float
Code unit for time in units of seconds.
- unit_m: float
Code unit for mass in units of gram.
- __init__(gamma=1.4, x0=0.5, rhoL=1.0, rhoR=0.125, PL=1.0, PR=0.1, uL=0.0, uR=0.0, unit_l=1.0, unit_t=1.0, unit_m=1.0)
Free-fall
The image below shows the free-fall test at the initial and two later times:
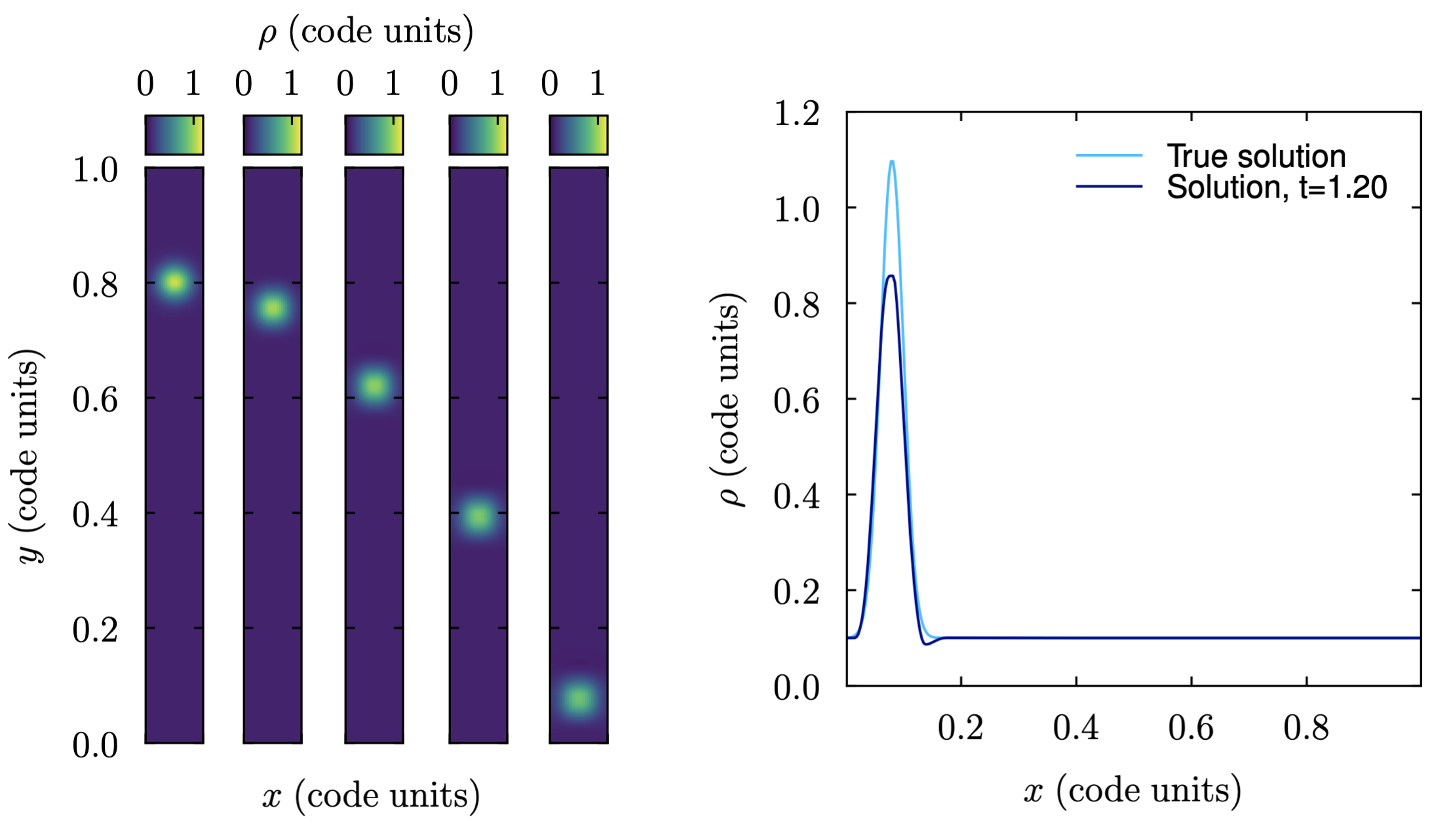
- class ulula.setups.freefall.SetupFreefall(unit_l=1.0, unit_t=1.0, unit_m=1.0)
Gravitational free-fall
This setup is mostly a test of the gravity solver. The outflow BCs mean that the entire domain is free-falling under a constant gravitational acceleration. We compare the position of a Gaussian gas blob in pressure equilibrium to the known solution. This setup demonstrates
Fixed-acceleration gravity combined with outflow boundary conditions
Accuracy of the gravity source terms.
- Parameters:
- unit_l: float
Code unit for length in units of centimeters.
- unit_t: float
Code unit for time in units of seconds.
- unit_m: float
Code unit for mass in units of gram.
- __init__(unit_l=1.0, unit_t=1.0, unit_m=1.0)
Atmosphere
The image below shows the atmosphere setup at the initial time and after 10 hours:
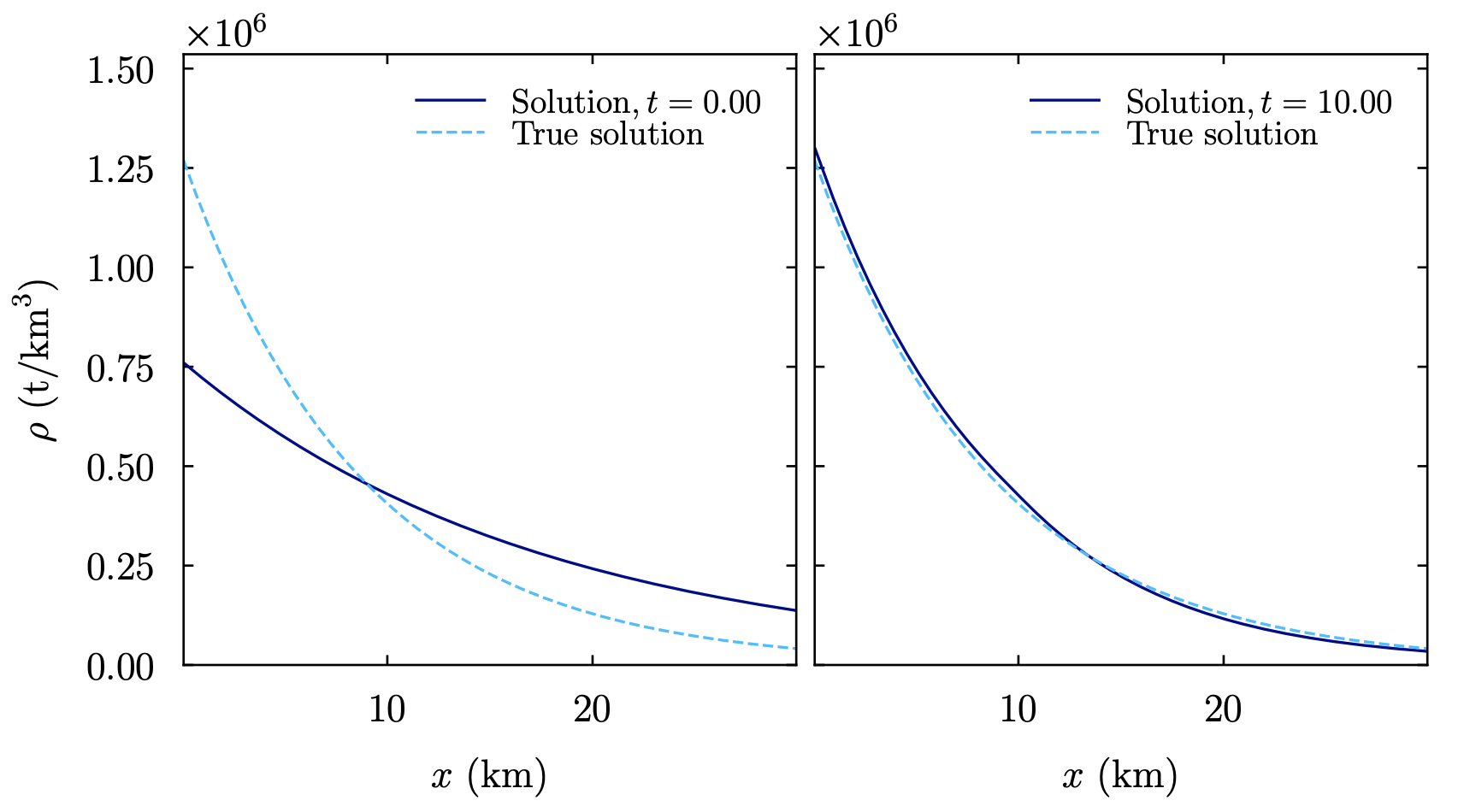
- class ulula.setups.atmosphere.SetupAtmosphere(unit_l=100000.0, unit_t=3600.0, unit_m=1000000000000.0, T_K=300.0)
Earth’s hydrostratic atmosphere
This setup represents the Earth’s atmosphere in 1D, which settles into an exponential density and pressure profile (for an isothermal equation of state). The initial density is perturbed away from the true solution by assuminh a “wrong” scale height. After some time, it settles to the correct solution. By default, the code units are set to kilometers, hours, and tons. This setup demonstrates
Fixed-acceleration gravity with wall boundary conditions
Isothermal equation of state
Code units suitable to Earth conditions, including Earth gravity.
Depending on the initial conditions, this setup can be numerically unstable due to very strong motions that develop into shocks. For example, if too much mass is initially placed in the upper atmosphere, that mass falls to the surface. Similarly, extending the upper limit to much higher altitude than the default 30 km can lead to difficulties due to the very low pressure and density.
- Parameters:
- unit_l: float
Code unit for length in units of centimeters.
- unit_t: float
Code unit for time in units of seconds.
- unit_m: float
Code unit for mass in units of gram.
- T_K: float
Air temperature in Kelvin.
- __init__(unit_l=100000.0, unit_t=3600.0, unit_m=1000000000000.0, T_K=300.0)